If you are a developer or a web dev enthusiast, the following thought of exasperation might have crossed your mind a couple of times or more;
“Wait… I have to build the admin dashboard too?”
If you’ve ever sighed dramatically at the thought of building an admin interface from scratch, writing repetitive CRUD code, styling every table row, and chasing edge-case bugs in form validation, then buddy, Laravel Filament might just be your new favorite sidekick.
We get it. As a Laravel developer, your dream probably isn’t to handcraft yet another admin panel.
You want something modern, flexible, secure, and, dare we say, enjoyable to work with.
Well, Filament isn’t just another admin panel. It’s a developer-first framework designed to help you build elegant admin interfaces (and even public-facing apps) with speed, style, and zero JavaScript stress.
Whether you’re building an internal dashboard, CMS, or SaaS backend, we at Mavlers with a rich experience of 13+ years in the web dev field, will walk you through everything you need to know about Laravel Filament, what makes it awesome, how it works under the hood, and why it might just be the best thing to happen to your Laravel workflow since Livewire.
On that note, let’s get down to it!
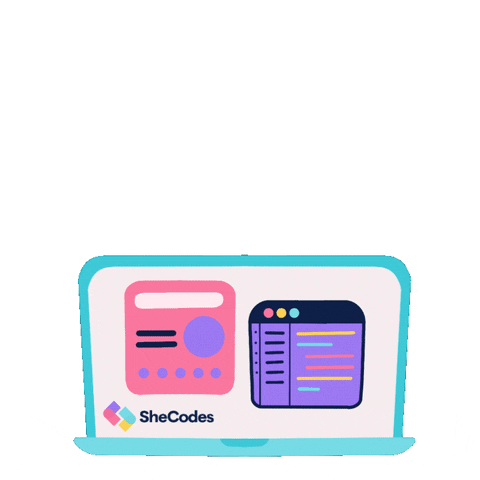
So, what exactly is Laravel Filament?
At its core, Laravel Filament is a modern, reactive admin panel framework built on top of Laravel Livewire. It comes with a beautifully designed UI powered by Tailwind CSS and takes full advantage of Laravel’s Eloquent ORM to make backend operations feel like a breeze.
One can think of it like this: Filament is the glue between robust Laravel features and a gorgeous, responsive admin interface without writing a single line of JavaScript.
And if you’re already familiar with Laravel and Livewire, using Filament will feel like slipping into your favorite hoodie: familiar, cozy, and incredibly productive.
Under the hood: Understanding the core architecture
Before we dive into the magic, let’s take a peek at the ingredients that make Filament tick:
- Livewire foundation: This enables reactive, dynamic interfaces without JavaScript.
- Tailwind CSS: Proffers utility-first, clean, and fully responsive styling.
- Alpine.js: Adds interactivity (client-side interactions) when Livewire needs a hand.
- Eloquent: It offers deep integration with Laravel’s ORM, which roughly translates into database magic with minimal effort.
In short, Filament leverages all the tools you already love and blends them into something truly seamless.
An insight into the features that make Filament a thorough game changer
To be honest, admin panels usually involve a lot of tedious repetition. Filament steals the show by flipping that on its head and giving you a powerful, intuitive toolkit that feels like Laravel’s artisan commands had a glow-up.
1. Revolutionary Resource Management System
When you work with Filament Resources, building CRUD interfaces becomes ridiculously simple.
php artisan make:filament-resource Product
This one liner generates;
- A model (if you don’t already have one)
- A form schema for creating/editing
- A table schema for listing records
- Authorization policies
…all wired together and ready to go. It’s basically CRUD on autopilot.
2. Beautifully flexible Form Builder
Do you need to build smart, interactive forms? Filament’s form builder has your back with field validation, dynamic behaviors, and layout controls baked in.
Forms\Components\Section::make('Product Details')
->schema([
Forms\Components\TextInput::make('name')
->required()
->maxLength(255)
->live(onBlur: true)
->afterStateUpdated(function ($state, $set) {
$set('slug', Str::slug($state));
}),
Forms\Components\TextInput::make('slug')
->required()
->unique(ignoreRecord: true),
Forms\Components\RichEditor::make('description')
->columnSpanFull(),
Forms\Components\FileUpload::make('image')
->image()
->directory('products')
->preserveFilenames(),
])
->columns(2)
Need to create slugs from names on blur? Done.
Want to set field-level uniqueness? Yup.
Do you have to upload images and preserve file names? You bet.
3. An advanced table builder that rivals premium UI kits
Filament’s table builder doesn’t just show data; it lets you interact with it. Think:
- Searchable, sortable columns
- Toggleable timestamps
- Circular image previews
- Currency formats
- Boolean badges
- Even inline actions with confirmation prompts
Tables\Columns\ImageColumn::make('image')
->circular()
->defaultImageUrl(url('/default-product.png')),
Tables\Columns\TextColumn::make('name')
->searchable()
->sortable()
->description(fn (Product $record) => $record->category?->name),
Tables\Columns\IconColumn::make('is_active')
->boolean()
->label('Active'),
Tables\Columns\TextColumn::make('price')
->money('USD')
->sortable(),
Tables\Columns\TextColumn::make('created_at')
->dateTime()
->sortable()
->toggleable(isToggledHiddenByDefault: true)
What sets Filament apart from other starter kits?
So why pick Filament over other admin starters like Laravel Nova, Voyager, or hand-rolled UIs? Glad you asked. Here’s why!
- Built for developers by developers!
If Laravel is your jam, Filament speaks your language quite fluently.
- Chained methods: Its fluent API feels like Laravel’s query builder
- Hot reloading: Offers instant updates during development
- IDE-friendly: Comes with stellar autocompletion and type hints
- Built-in testing support: Proffers easy integration with Pest and PHPUnit
2. The perfect confluence of speed and performance
Filament isn’t just pretty; it’s also fast. Replete with the following features, it packs quite a punch!
- Lazy Loading: Only fetches what’s needed
- Smart DOM Rendering: Fewer updates = faster response
- Query Optimization: Automatic eager loading prevention
- Caching: Built-in strategies to minimize redundant queries
The result is real-world performance that scales.
3. Seamless ecosystem integration
Filament takes to other tools in the ecosystem like fish to water;
- Spatie packages (Media Library, Roles/Permissions, Tags)
- Stripe / PayPal for payment modules
- Vue components if you need to sprinkle in extra interactivity
- Even Laravel Nova resources can be ported over
Show us the real dirt, honey! Delving into real use cases
- Enterprise admin panels
Filament shines in complex business environments such as multi-tenancy support, approval workflows, audit trails, and reporting dashboards.
Here’s an example of a workflow builder;
Tables\Actions\Action::make('approve')
->requiresConfirmation()
->action(function (Post $post) {
$post->update(['status' => 'approved']);
Notification::make()
->title('Post approved')
->success()
->send();
})
->visible(fn (Post $post) => $post->status === 'pending')
2. Content management system
Guess what? You can even build a WordPress-style block editor with Filament:
// Custom block editor
Forms\Components\Builder::make('content')
->blocks([
Forms\Components\Builder\Block::make('heading')
->schema([
Forms\Components\TextInput::make('content')
->label('Heading')
->required(),
Forms\Components\Select::make('level')
->options([
'h1' => 'Heading 1',
'h2' => 'Heading 2',
// ...
]),
]),
Forms\Components\Builder\Block::make('paragraph')
->schema([
Forms\Components\RichEditor::make('content')
->label('Paragraph')
->required(),
]),
])
3. SaaS app backends
For SaaS products, Filament provides user management, subscription handling, feature flagging and analytics integration.
Example of subscription management: The following code shows a table column with a subscription and action to be taken on the column.
Tables\Columns\TextColumn::make('subscription.status')
->badge()
->color(fn (string $state): string => match ($state) {
'active' => 'success',
'canceled' => 'danger',
'past_due' => 'warning',
default => 'gray',
}),
Tables\Actions\Action::make('cancelSubscription')
->requiresConfirmation()
->action(function (User $user) {
$user->subscription->cancel();
})
->visible(fn (User $user) => $user->subscription?->active())
An insight into the advanced stuff you can do with Filament
Filament goes beyond CRUD and can also help you with;
- Custom themes: To match your brand’s look and feel
- Plugin ecosystem: To extend or share components
- API layers: To build API-first applications with ease
When should you use (or skip) Filament?
You want to know whether you should go for it or steer clear. If you are still on the fence, here’s how to decide.
Say yaaay if you have;
- Rapid prototypes
- Internal dashboards
- Content-heavy sites (blogs, docs)
- Medium-complexity SaaS products
Say nay if you have;
- Ultra-custom frontends with heavy JS (use Inertia/Vue instead)
- Public-facing high-performance sites
- Design systems that break the “admin pattern” mold
Exploring performance benchmarks (because you asked!)
In comparative tests against similar solutions, here’s how Filament fared:
- Memory usage: 15-20% lower than traditional admin panels
- Initial load: 30% faster due to optimized asset delivery
- CRUD operations: Comparable to hand-optimized controllers
- Scalability: Handles 10k+ records comfortably with proper indexing
Gazing into the crystal ball: Decoding the future for Filament
Filament’s roadmap looks bright and promising. Here’s a glimpse of what lies at the end of the tunnel;
- Better mobile support
- Deeper GraphQL integrations
- Improved i18n (localization)
- AI-powered helpers for faster scaffolding
When asked about why Filament is so transformative, here’s what our web dev ninja, Hardik Kotak, had to say;
“Laravel Filament represents a paradigm shift in admin panel development by:
- Eliminating boilerplate: Reduces repetitive CRUD code by 80%+
- Enforcing best practices: Offers built-in solutions for common challenges
- Maintaining flexibility: Come up with escape hatches for custom requirements
- Accelerating development: Projects that took weeks now take days
For Laravel developers, Filament isn’t just another admin panel; it’s a complete application framework that changes how we approach backend development, allowing us to focus on business logic rather than repetitive interface code.”
The road ahead
If you are a fan of all things related to Laravel, you might want to explore ~ Laravel 12: An Early Look at the Future of Web Development.
Hardik Kotak
Hardik is a seasoned Subject Matter Expert (SME) and Team Lead at Mavlers, with over 13 years of experience in the tech industry. With a strong background in full-stack web application development, he specializes in using Laravel, HTML, CSS (Bootstrap), and PHP to create robust solutions. His expertise also extends to developing APIs for mobile applications and managing server infrastructure on AWS.
Naina Sandhir - Content Writer
A content writer at Mavlers, Naina pens quirky, inimitable, and damn relatable content after an in-depth and critical dissection of the topic in question. When not hiking across the Himalayas, she can be found buried in a book with spectacles dangling off her nose!
The Marketer’s Guide to Rising Google Ads CPC: Causes, Fixes, and Future-Proof Strategies
Velocity Scripting in Marketo: A Pain-free Intro, Methodology, Use Cases